3.2. Managing S3 Users in WHMCS¶
This section describes how to manage users in WHMCS in a service provider scenario. New customers will sign up for the service during purchase in your online store and you will need to create users for them in the S3 cluster.
Create all files mentioned further in the directory whmcs/admin/AcronisStorage
.
3.2.1. Creating S3 Users¶
You can create a user with the ostor-users
service and parameter emailAddress
specifying the user email address. WHMCS creates the user in S3 cluster when you click Create User. Create a file S3_createUser.php
with the following contents:
<?php
// Load configuration and libraries.
require('../../includes/AcronisStorage/S3_addClientNote.php');
require('../../includes/AcronisStorage/S3_getClient.php');
require('../../includes/AcronisStorage/S3_getConfig.php');
require('../../includes/AcronisStorage/S3_requestCurl.php');
require('../../init.php');
// Create s3 user.
function S3_createUser($userid) {
// Load configuration.
$s3_config = s3_getConfig();
// Get whmcs user email.
$s3_whmcs = S3_getClient($userid, $s3_config['whmcs_username']);
// Create s3 user.
$s3_client = S3_requestCurl(
$s3_config['s3_key'],
$s3_config['s3_secret'],
$s3_config['s3_gateway'],
"/?ostor-users&emailAddress=" . $s3_whmcs['email'],
"PUT"
);
// Add note with the s3 access key and s3 secret.
S3_addClientNote(
$s3_whmcs['userid'],
$s3_config['whmcs_username'],
$s3_client['UserId'],
$s3_client['AWSAccessKeys']['0']['AWSAccessKeyId'],
$s3_client['AWSAccessKeys']['0']['AWSSecretAccessKey']
);
// Redirect back.
header('Location: ' . $_SERVER['HTTP_REFERER']);
}
// Call function.
S3_createUser($_GET['userid']);
?>
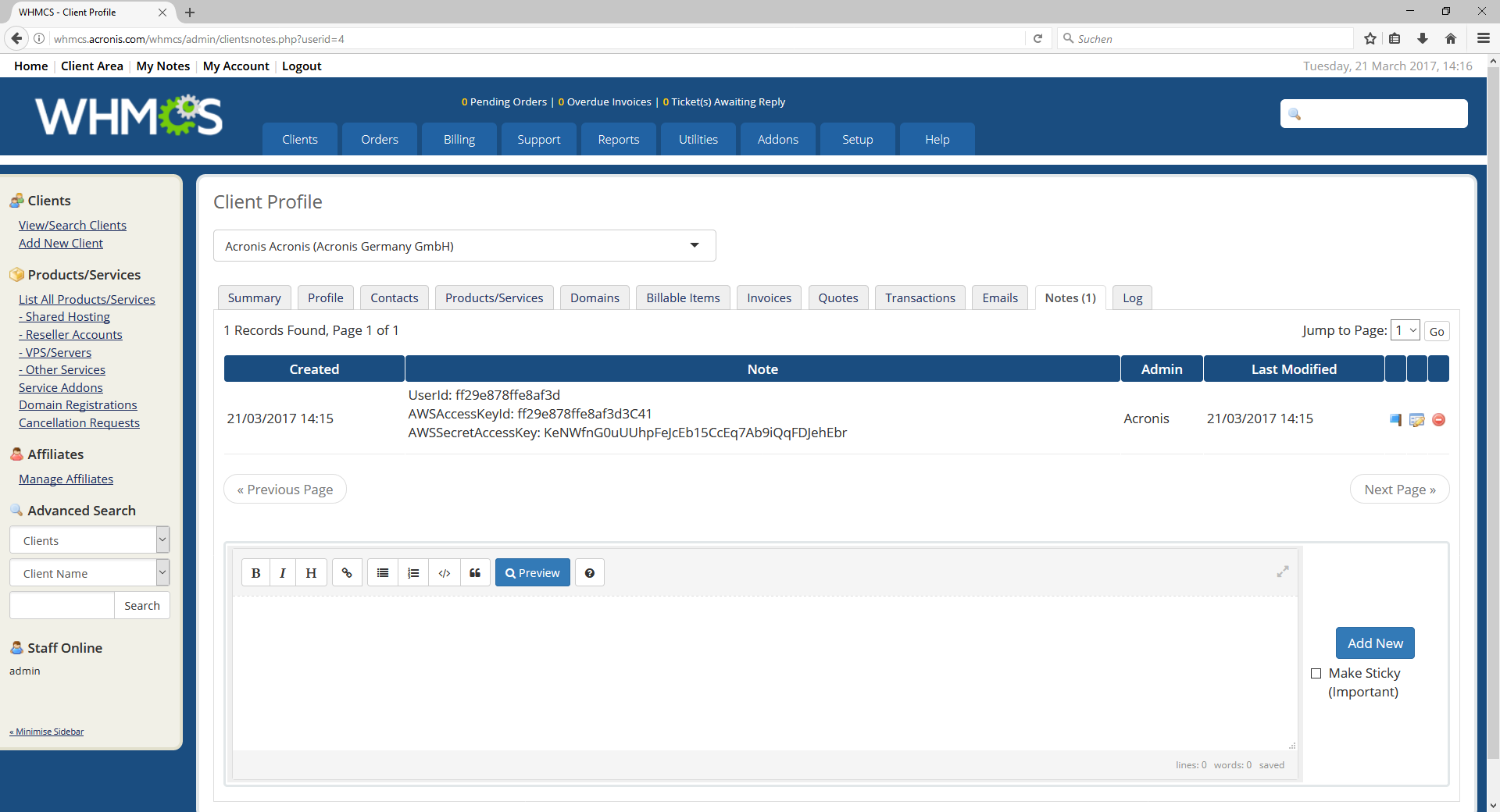
3.2.2. Listing S3 Users¶
You can list information about all users with the ostor-users
service. Additional rows may list S3 access key pairs associated with the user. WHMCS lists the users information fetched from S3 cluster when you click List Users (on/off). Create a file S3_listUsers.php
with the following contents:
<?php
// Load configuration and libraries.
require('../../includes/AcronisStorage/S3_getConfig.php');
require('../../includes/AcronisStorage/S3_requestCurl.php');
require('../../init.php');
// List s3 users.
function S3_listUsers() {
// Hide now.
if ($_SESSION['s3_list_users'] == 1) {
// Hide.
$_SESSION['s3_list_users'] = 0;
// Redirect back.
header('Location: ' . $_SERVER['HTTP_REFERER']);
// Return immediately.
return;
}
// Load configuration.
$s3_config = s3_getConfig();
// Get s3 users.
$s3_client = S3_requestCurl(
$s3_config['s3_key'],
$s3_config['s3_secret'],
$s3_config['s3_gateway'],
"/?ostor-users",
"GET"
);
// Store s3 result.
$_SESSION['s3_list_users'] = 1;
$_SESSION['s3_list'] = $s3_client;
// Redirect back.
header('Location: ' . $_SERVER['HTTP_REFERER']);
}
// Call function.
S3_listUsers();
?>
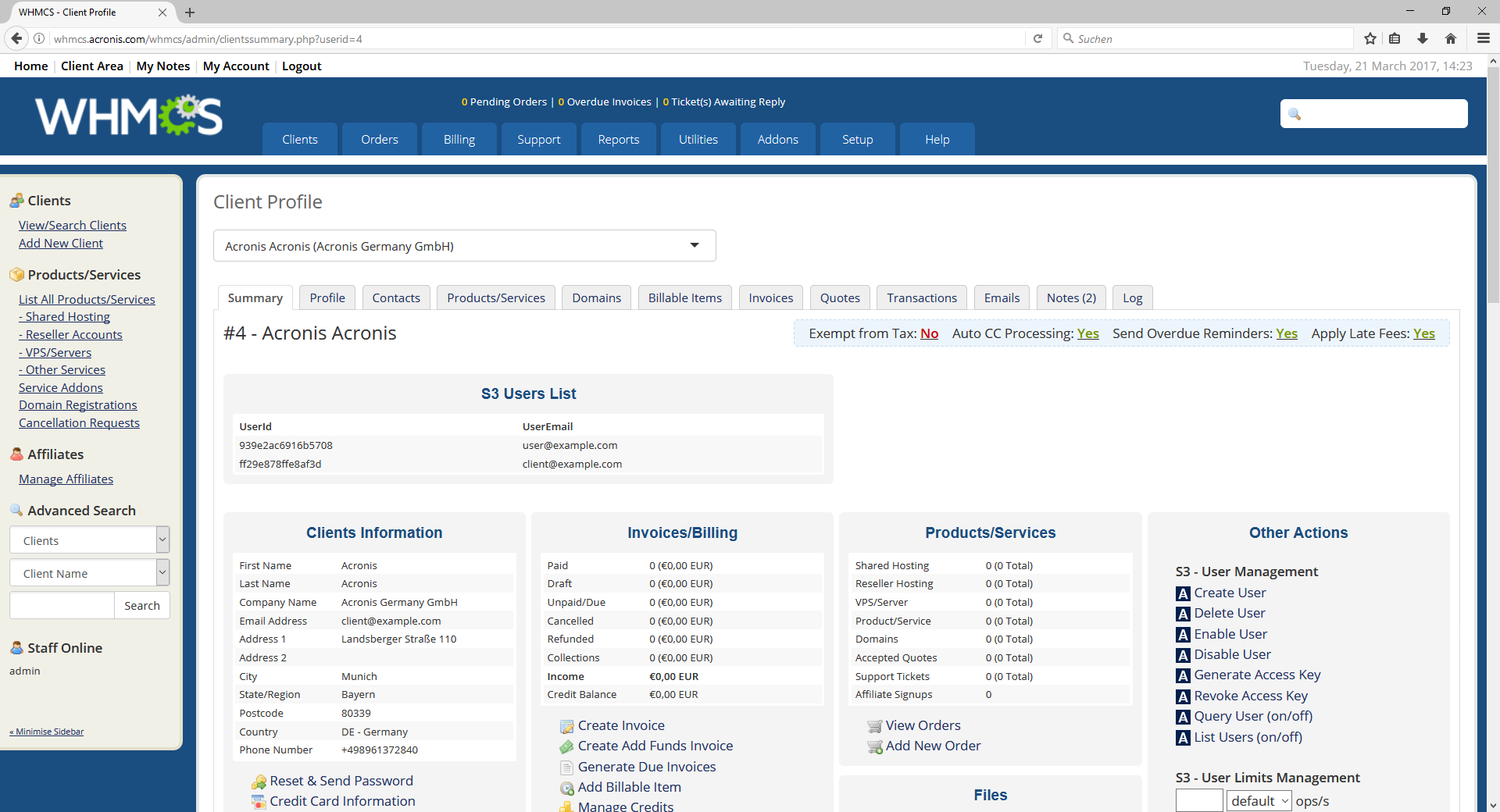
3.2.3. Querying S3 Users¶
You can display information and status of a user with the ostor-users
service and parameter emailAddress
specifying the user email address. WHMCS displays the user information fetched from S3 cluster when you click Query User (on/off). Create a file S3_queryUser.php
with the following contents:
<?php
// Load configuration and libraries.
require('../../includes/AcronisStorage/S3_getClient.php');
require('../../includes/AcronisStorage/S3_getConfig.php');
require('../../includes/AcronisStorage/S3_requestCurl.php');
require('../../init.php');
// Query s3 user.
function S3_queryUser($userid) {
// Hide now.
if ($_SESSION['s3_query_user'] == 1) {
// Hide.
$_SESSION['s3_query_user'] = 0;
// Redirect back.
header('Location: ' . $_SERVER['HTTP_REFERER']);
// Return immediately.
return;
}
// Load configuration.
$s3_config = s3_getConfig();
// Get whmcs user email.
$s3_whmcs = S3_getClient($userid, $s3_config['whmcs_username']);
// Get s3 user id.
$s3_client = S3_requestCurl(
$s3_config['s3_key'],
$s3_config['s3_secret'],
$s3_config['s3_gateway'],
"/?ostor-users&emailAddress=" . $s3_whmcs['email'],
"GET"
);
// Store s3 result.
$_SESSION['s3_query_user'] = 1;
$_SESSION['s3_userid'] = $s3_client['UserId'];
$_SESSION['s3_aws_access_keys'] = $s3_client['AWSAccessKeys'];
// Redirect back.
header('Location: ' . $_SERVER['HTTP_REFERER']);
}
// Call function.
S3_queryUser($_GET['userid']);
?>
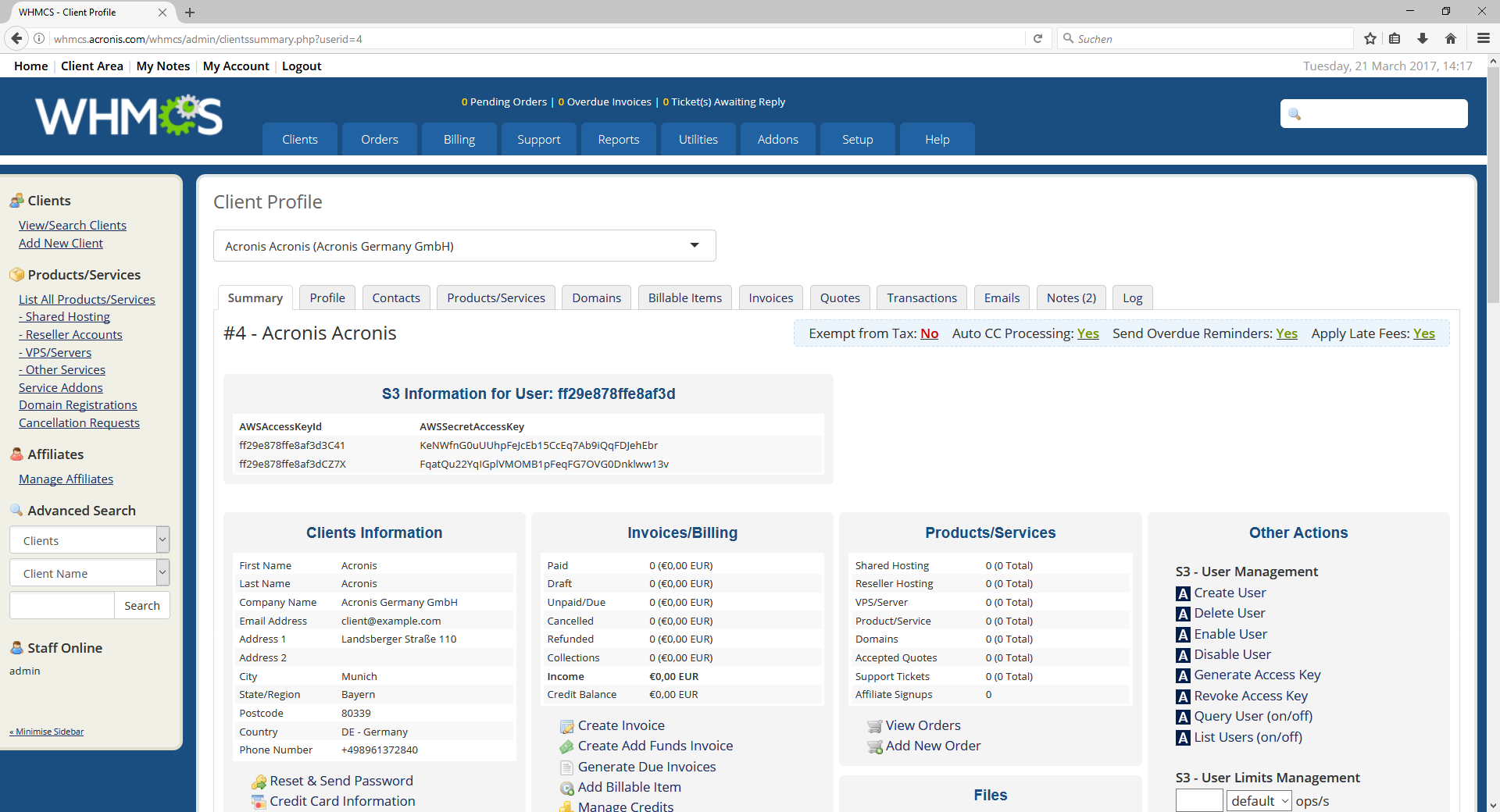
3.2.4. Disabling S3 Users¶
You can disable users with the ostor-users
service and parameter emailAddress
specifying the user email address. WHMCS disables read and write access to S3 cluster when you click Disable User. Create a file S3_disableUser.php
with the following contents:
<?php
// Load configuration and libraries.
require('../../includes/AcronisStorage/S3_getClient.php');
require('../../includes/AcronisStorage/S3_getConfig.php');
require('../../includes/AcronisStorage/S3_requestCurl.php');
require('../../init.php');
// Disable user.
function S3_disableUser($userid) {
// Load configuration.
$s3_config = s3_getConfig();
// Get whmcs user email.
$s3_whmcs = S3_getClient($userid, $s3_config['whmcs_username']);
// Disable user.
$s3_client = S3_requestCurl(
$s3_config['s3_key'],
$s3_config['s3_secret'],
$s3_config['s3_gateway'],
"/?ostor-users&emailAddress=" . $s3_whmcs['email'] . "&disable",
"POST"
);
// Redirect back.
header('Location: ' . $_SERVER['HTTP_REFERER']);
}
// Call function.
S3_disableUser($_GET['userid']);
?>
3.2.5. Enabling S3 Users¶
You can enable a previously disabled user with the ostor-users
service and parameter emailAddress
specifying the user email address. WHMCS enables read and write access to S3 cluster for user when you click Enable User. Create a file S3_enableUser.php
with the following contents:
<?php
// Load configuration and libraries.
require('../../includes/AcronisStorage/S3_getClient.php');
require('../../includes/AcronisStorage/S3_getConfig.php');
require('../../includes/AcronisStorage/S3_requestCurl.php');
require('../../init.php');
// Enable user.
function S3_enableUser($userid) {
// Load configuration.
$s3_config = s3_getConfig();
// Get whmcs user email.
$s3_whmcs = S3_getClient($userid, $s3_config['whmcs_username']);
// Enable user.
$s3_client = S3_requestCurl(
$s3_config['s3_key'],
$s3_config['s3_secret'],
$s3_config['s3_gateway'],
"/?ostor-users&emailAddress=" . $s3_whmcs['email'] . "&enable",
"POST"
);
// Redirect back.
header('Location: ' . $_SERVER['HTTP_REFERER']);
}
// Call function.
S3_enableUser($_GET['userid']);
?>
3.2.6. Deleting S3 Users¶
You can delete users with the ostor-users
service and parameter emailAddress
specifying the user email address. WHMCS removes the user from S3 cluster when you click Delete User. Create a file S3_deleteUser.php
with the following contents:
<?php
// Load configuration and libraries.
require('../../includes/AcronisStorage/S3_delClientNote.php');
require('../../includes/AcronisStorage/S3_getClient.php');
require('../../includes/AcronisStorage/S3_getConfig.php');
require('../../includes/AcronisStorage/S3_requestCurl.php');
require('../../init.php');
// Delete s3 user.
function S3_deleteUser($userid) {
// Load configuration.
$s3_config = s3_getConfig();
// Get whmcs user email.
$s3_whmcs = S3_getClient($userid, $s3_config['whmcs_username']);
// Get s3 user id.
$s3_client = S3_requestCurl(
$s3_config['s3_key'],
$s3_config['s3_secret'],
$s3_config['s3_gateway'],
"/?ostor-users&emailAddress=" . $s3_whmcs['email'],
"GET"
);
// Delete s3 user.
S3_requestCurl(
$s3_config['s3_key'],
$s3_config['s3_secret'],
$s3_config['s3_gateway'],
"/?ostor-users&emailAddress=" . $s3_whmcs['email'],
"DELETE"
);
// Delete note with the s3 access key and s3 secret.
S3_delClientNote(
$s3_whmcs['userid'],
$s3_config['whmcs_username'],
$s3_client['UserId'],
""
);
// Redirect back.
header('Location: ' . $_SERVER['HTTP_REFERER']);
}
// Call function.
S3_deleteUser($_GET['userid']);
?>
3.2.7. Generating S3 Access Keys¶
You can generate a new or additional access key pair with the ostor-users
service and the following parameters: emailAddress
specifying the user email address, genKey
. WHMCS generates a new key pair when you click Generate Access Key. Create a file S3_generateAccessKey.php
with the following contents:
<?php
// Load configuration and libraries.
require('../../includes/AcronisStorage/S3_addClientNote.php');
require('../../includes/AcronisStorage/S3_getClient.php');
require('../../includes/AcronisStorage/S3_getConfig.php');
require('../../includes/AcronisStorage/S3_requestCurl.php');
require('../../init.php');
// Generate s3 access key pair.
function S3_generateAccessKey($userid) {
// Load configuration.
$s3_config = s3_getConfig();
// Get whmcs user email.
$s3_whmcs = S3_getClient($userid, $s3_config['whmcs_username']);
// Generate s3 key pair.
$s3_client = S3_requestCurl(
$s3_config['s3_key'],
$s3_config['s3_secret'],
$s3_config['s3_gateway'],
"/?ostor-users&emailAddress=" . $s3_whmcs['email'] . "&genKey",
"POST"
);
// Add note with the s3 access key and s3 secret.
S3_addClientNote(
$s3_whmcs['userid'],
$s3_config['whmcs_username'],
$s3_client['UserId'],
$s3_client['AWSAccessKeys']['0']['AWSAccessKeyId'],
$s3_client['AWSAccessKeys']['0']['AWSSecretAccessKey']
);
// Redirect back.
header('Location: ' . $_SERVER['HTTP_REFERER']);
}
// Call function.
S3_generateAccessKey($_GET['userid']);
?>
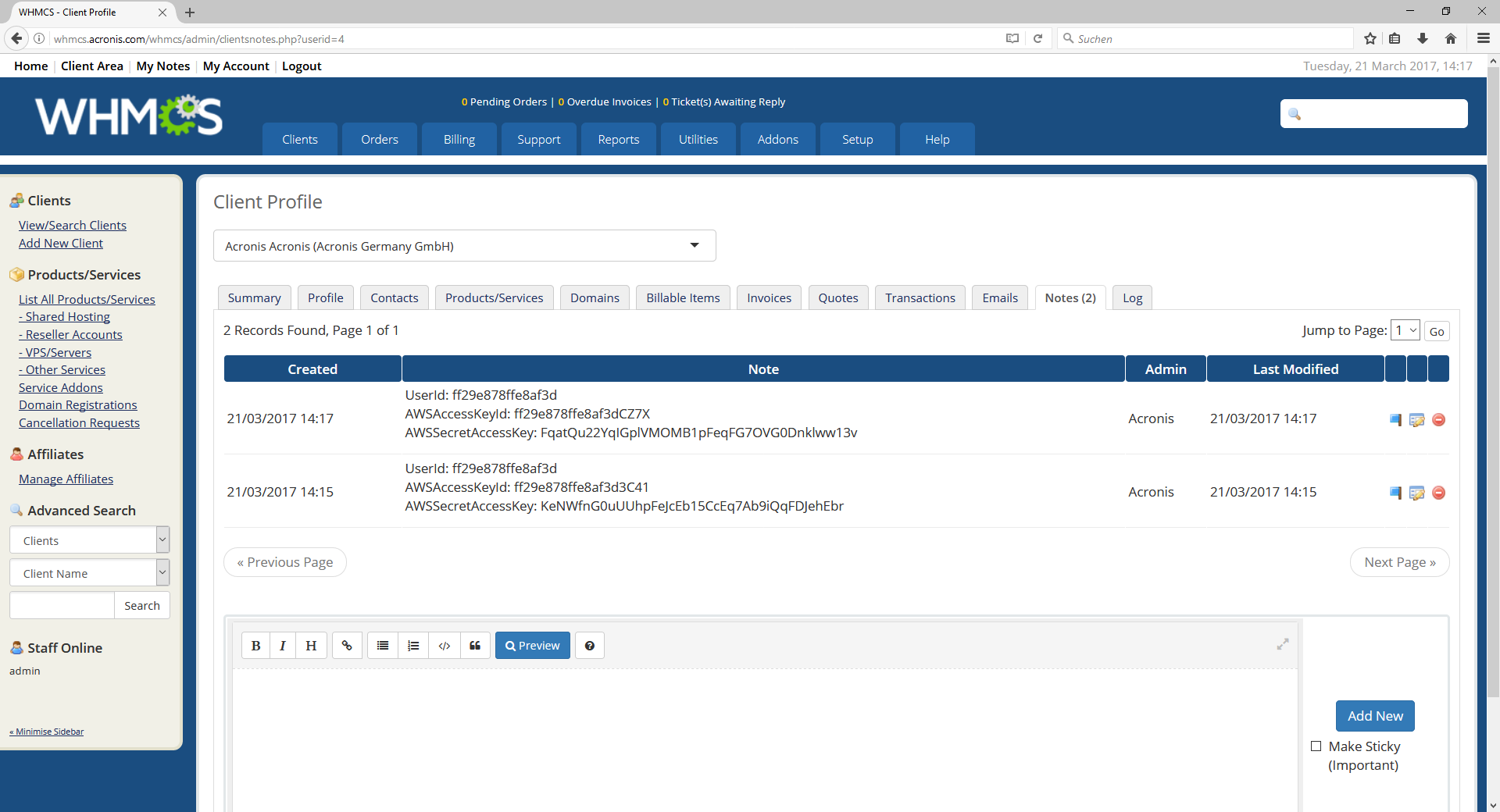
3.2.8. Revoking S3 Access Keys¶
You can revoke the specified access key pair of the specified user with the ostor-users
service and the following parameters: emailAddress
specifying the user email address, revokeKey
specifying the access key in the key pair. WHMCS removes the key pair when you click Revoke Access Key. Create a file S3_revokeAccessKey.php
with the following contents:
<?php
// Load configuration and libraries.
require('../../includes/AcronisStorage/S3_delClientNote.php');
require('../../includes/AcronisStorage/S3_getClient.php');
require('../../includes/AcronisStorage/S3_getConfig.php');
require('../../includes/AcronisStorage/S3_requestCurl.php');
require('../../init.php');
// Revoke s3 access key pair.
function S3_revokeAccessKey($userid) {
// Load configuration.
$s3_config = s3_getConfig();
// Get whmcs user email.
$s3_whmcs = S3_getClient($userid, $s3_config['whmcs_username']);
// Get first s3 access key.
$s3_client = S3_requestCurl(
$s3_config['s3_key'],
$s3_config['s3_secret'],
$s3_config['s3_gateway'],
"/?ostor-users&emailAddress=" . $s3_whmcs['email'],
"GET"
);
// Revoke s3 access key.
S3_requestCurl(
$s3_config['s3_key'],
$s3_config['s3_secret'],
$s3_config['s3_gateway'],
"/?ostor-users&emailAddress=" . $s3_whmcs['email'] .
"&revokeKey=" . $s3_client['AWSAccessKeys']['0']['AWSAccessKeyId'],
"POST"
);
// Delete note with the s3 access key and s3 secret.
S3_delClientNote(
$s3_whmcs['userid'],
$s3_config['whmcs_username'],
$s3_client['UserId'],
$s3_client['AWSAccessKeys']['0']['AWSAccessKeyId']
);
// Redirect back.
header('Location: ' . $_SERVER['HTTP_REFERER']);
}
// Call function.
S3_revokeAccessKey($_GET['userid']);
?>